Debugging frontend code is an essential skill for web developers. Understanding and mastering various debugging techniques can significantly improve your ability to identify and resolve issues quickly. In this article, we will explore two fundamental and powerful methods of debugging frontend code: using console.log
and employing breakpoints/debugger
.
console.log
One of the simplest yet most effective ways to debug frontend code is by using console.log
. this method allow developers to print information to the browser’s console, providing insight into the application’s state and behavior.
Using console.log
The console.log
method prints any specified message or variable to the console. It’s incredibly versatile and can be used to display strings, numbers, arrays, objects, and more.
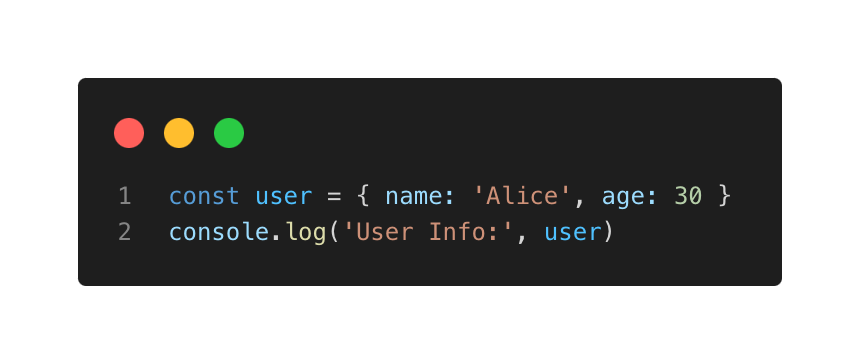
The output provides a collapsible list of the object’s properties, allowing you to drill down into nested objects and arrays.:

Benefits of console.log
- Immediate Feedback: Quickly see the values of variables and the flow of code execution.
- Ease of Use: Simple to implement with minimal setup.
- Versatility: Effective for logging different data types and structures.
However, relying solely on console.log
for debugging can lead to cluttered code and missed breakpoints. This is where breakpoints and the debugger
statement come into play.
Breakpoints and debugger
Breakpoints and the debugger
statement offer a more sophisticated approach to debugging by allowing you to pause code execution and inspect the program's state at specific points.
Using Breakpoints
Modern browsers come equipped with developer tools that allow you to set breakpoints in your code. Breakpoints pause the execution at a specific line, enabling you to inspect variables, evaluate expressions, and step through code line by line.
To set a breakpoint in Chrome:
- Open Developer Tools (F12 or right-click and select "Inspect").
- Go to the "Sources" panel.
- Navigate to your JavaScript file and click on the line number where you want to set the breakpoint.
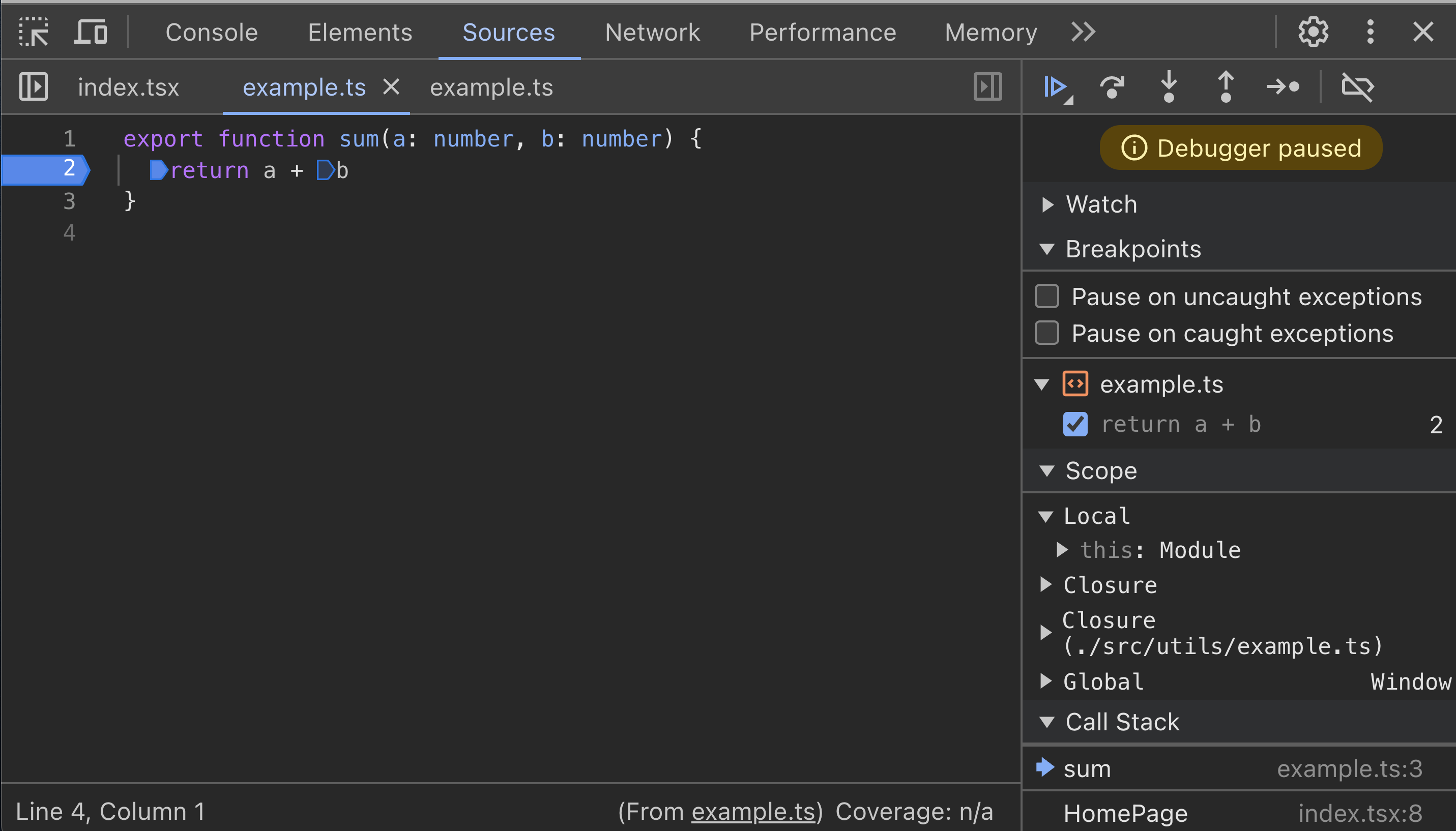
Once the breakpoint is hit, you can:
- Inspect Variables: Hover over variables to see their current values.
- Evaluate Expressions: Use the watch to run JavaScript expressions in the context of the paused execution.
- Step Through Code: Use the controls to step over, into, or out of functions to understand the execution flow.

Using the debugger
Statement
The debugger
statement acts like a manual breakpoint. When the JavaScript engine encounters this statement, it pauses execution and brings up the debugging interface.

When this code runs, the debugger will pause execution at the debugger
statement, allowing you to inspect the state at that exact point.
Benefits of Breakpoints and debugger
- Precise Control: Pause execution exactly where needed and inspect the state in detail.
- Flow Analysis: Step through the code to understand the sequence of operations and logic flow.
- Contextual Debugging: Evaluate the context in which a piece of code executes, which is crucial for understanding scope and closures.
Conclusion
Both console.log
and breakpoints/debugger
are indispensable tools in a web developer's debugging toolkit. console.log
offer quick and versatile logging, while breakpoints and the debugger
statement provide deeper insight and control over code execution. By mastering these techniques, developers can efficiently diagnose and fix frontend issues, leading to more robust and reliable applications.